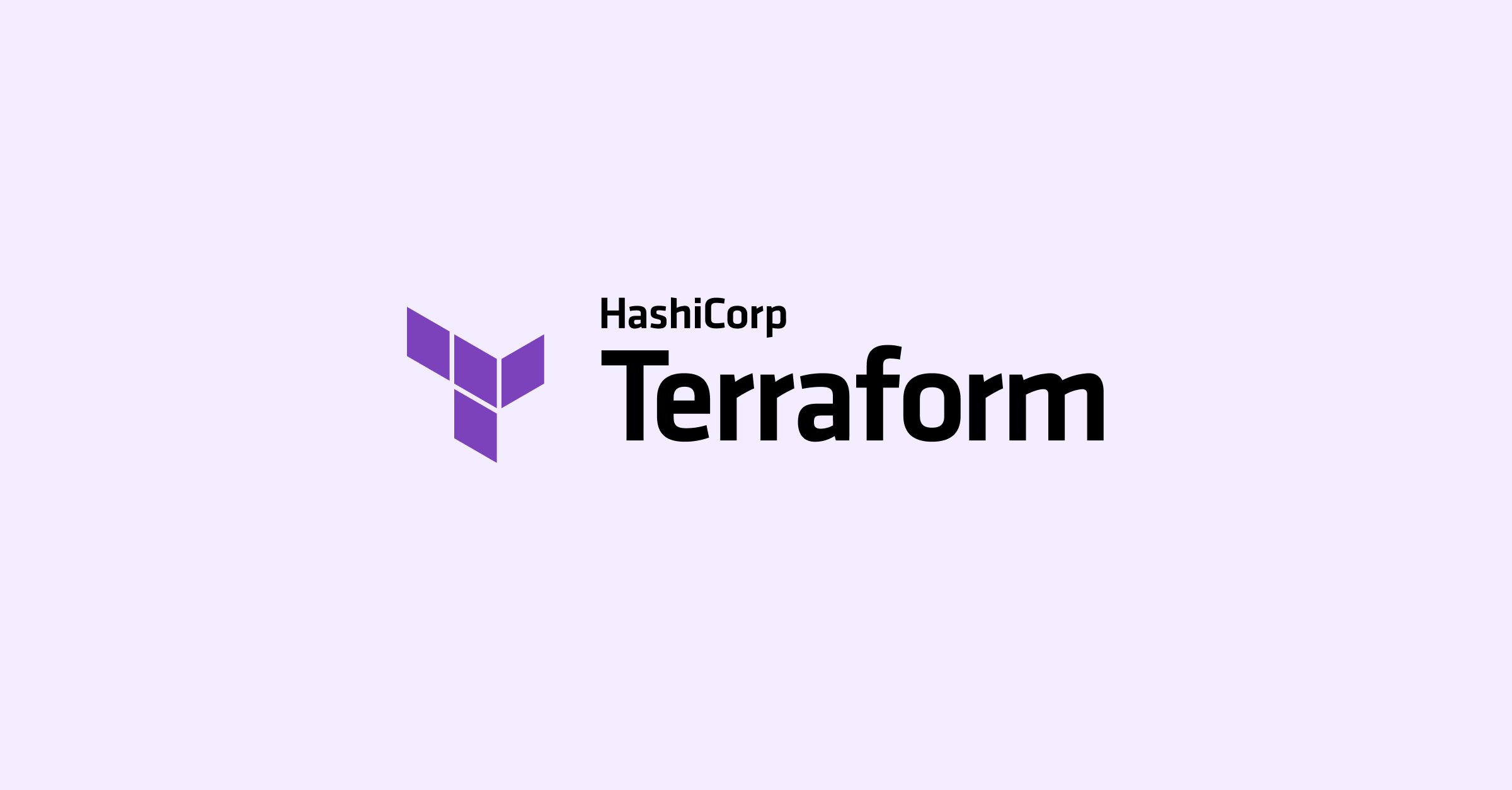
需求说明
在AWS上创建VPC,VPC启用三个可用区,每个可用区包含Private,Public subnet各一个,一个NAT Gateway,一个Internet Gateway
创建资源
新建 variables.tf
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53
| provider "aws" { region = var.region access_key = var.access_key secret_key = var.secret_key }
variable "region" { # 根据需要更改区域 type = string default = "xxx" description = "region" }
variable "access_key" { type = string default = "xxx" description = "access_key" }
variable "secret_key" { type = string default = "xxx" description = "secret_key" }
variable "vpc_cidr" { type = string default = "10.0.0.0/16" description = "vpc " }
variable "vpc_subnet_public1_cidr" { type = string default = "10.0.0.0/22" description = "vpc_subnet_public1_cidr" }
variable "vpc_subnet_public2_cidr" { type = string default = "10.0.4.0/22" description = "vpc_subnet_public2_cidr" }
variable "vpc_subnet_private1_cidr" { type = string default = "10.0.8.0/22" description = "vpc_subnet_private1_cidr" }
variable "vpc_subnet_private2_cidr" { type = string default = "10.0.12.0/22" description = "vpc_subnet_private2_cidr" }
|
新建vpc.tf
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130
| # 创建 VPC resource "aws_vpc" "vpc" { cidr_block = var.vpc_cidr enable_dns_support = true enable_dns_hostnames = true
tags = { Name = "vpc" } }
# 创建公有子网 resource "aws_subnet" "vpc_subnet_public1" { vpc_id = aws_vpc.vpc.id cidr_block = var.vpc_subnet_public1_cidr availability_zone = format("%s%s", var.region, "a") # 根据需要更改可用区
tags = { Name = "public_subnet_1a" } }
resource "aws_subnet" "vpc_subnet_public2" { vpc_id = aws_vpc.vpc.id cidr_block = var.vpc_subnet_public2_cidr availability_zone = format("%s%s", var.region, "b") # 根据需要更改可用区
tags = { Name = "public_subnet_2b" } }
# 创建私有子网 resource "aws_subnet" "vpc_subnet_private1" { vpc_id = aws_vpc.vpc.id cidr_block = var.vpc_subnet_private1_cidr availability_zone = format("%s%s", var.region, "a") # 根据需要更改可用区
tags = { Name = "private_subnet_2a" } }
resource "aws_subnet" "vpc_subnet_private2" { vpc_id = aws_vpc.vpc.id cidr_block = var.vpc_subnet_private2_cidr availability_zone = format("%s%s", var.region, "b") # 根据需要更改可用区
tags = { Name = "private_subnet_2b" } }
# 创建互联网网关 resource "aws_internet_gateway" "vpc_internet_gateway" { vpc_id = aws_vpc.vpc.id
tags = { Name = "igw" } }
# 创建 NAT 网关的弹性 IP resource "aws_eip" "vpc_nat_eip" { domain = "vpc" depends_on = [ aws_internet_gateway.vpc_internet_gateway ] }
# 创建 NAT 网关 resource "aws_nat_gateway" "vpc_nat_gateway" { allocation_id = aws_eip.vpc_nat_eip.id subnet_id = aws_subnet.vpc_subnet_public1.id # 公有子网中的任意一个 tags = { Name = "nat_gateway" } }
# 创建公有子网路由表 resource "aws_route_table" "vpc_public_router_table" { vpc_id = aws_vpc.vpc.id
route { cidr_block = "0.0.0.0/0" gateway_id = aws_internet_gateway.vpc_internet_gateway.id }
tags = { Name = "public_route_table" } }
# 将公有子网关联到公有子网路由表 resource "aws_route_table_association" "public_subnet_1" { subnet_id = aws_subnet.vpc_subnet_public1.id route_table_id = aws_route_table.vpc_public_router_table.id }
resource "aws_route_table_association" "public_subnet_2" { subnet_id = aws_subnet.vpc_subnet_public2.id route_table_id = aws_route_table.vpc_public_router_table.id }
# 创建私有子网路由表 resource "aws_route_table" "vpc_private_router_table" { vpc_id = aws_vpc.vpc.id
route { cidr_block = "0.0.0.0/0" nat_gateway_id = aws_nat_gateway.vpc_nat_gateway.id }
tags = { Name = "private_route_table" } }
# 将私有子网关联到私有子网路由表 resource "aws_route_table_association" "private_subnet_1" { subnet_id = aws_subnet.vpc_subnet_private1.id route_table_id = aws_route_table.vpc_private_router_table.id }
resource "aws_route_table_association" "private_subnet_2" { subnet_id = aws_subnet.vpc_subnet_private2.id route_table_id = aws_route_table.vpc_private_router_table.id }
|
运行
1 2 3 4 5
| terraform init # 查看预期变更 terraform plan # 生效变更 terraform apply
|